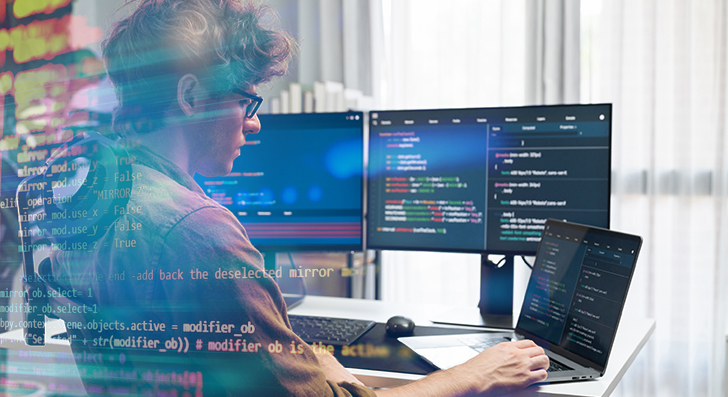
Scalability usually means your application can tackle expansion—far more consumers, more details, and much more visitors—without breaking. For a developer, creating with scalability in mind will save time and tension afterwards. Listed here’s a transparent and practical guide to assist you to start off by Gustavo Woltmann.
Style for Scalability from the beginning
Scalability isn't really one thing you bolt on afterwards—it should be element within your prepare from the beginning. A lot of applications fall short when they increase fast because the initial structure can’t manage the additional load. As being a developer, you'll want to Believe early regarding how your system will behave under pressure.
Start by planning your architecture to be versatile. Prevent monolithic codebases exactly where almost everything is tightly related. Rather, use modular style and design or microservices. These patterns break your app into scaled-down, unbiased components. Every single module or company can scale on its own without having impacting The entire technique.
Also, give thought to your database from day a single. Will it will need to take care of one million customers or perhaps 100? Pick the right sort—relational or NoSQL—determined by how your facts will mature. Plan for sharding, indexing, and backups early, even if you don’t require them but.
A further important stage is to prevent hardcoding assumptions. Don’t compose code that only performs beneath latest ailments. Contemplate what would materialize When your consumer base doubled tomorrow. Would your app crash? Would the database slow down?
Use design styles that aid scaling, like information queues or party-driven systems. These help your application cope with additional requests devoid of receiving overloaded.
If you Create with scalability in mind, you're not just preparing for success—you might be cutting down foreseeable future head aches. A effectively-planned procedure is simpler to keep up, adapt, and increase. It’s far better to prepare early than to rebuild afterwards.
Use the best Database
Choosing the ideal database is really a key Element of building scalable purposes. Not all databases are created exactly the same, and utilizing the Mistaken one can gradual you down and even cause failures as your application grows.
Commence by comprehension your information. Can it be very structured, like rows in a desk? If yes, a relational databases like PostgreSQL or MySQL is an effective in good shape. These are generally powerful with relationships, transactions, and regularity. They also assist scaling methods like examine replicas, indexing, and partitioning to handle additional site visitors and details.
Should your details is a lot more flexible—like consumer exercise logs, solution catalogs, or files—think about a NoSQL solution like MongoDB, Cassandra, or DynamoDB. NoSQL databases are much better at dealing with large volumes of unstructured or semi-structured knowledge and may scale horizontally additional effortlessly.
Also, take into account your read and compose styles. Are you undertaking many reads with fewer writes? Use caching and browse replicas. Are you handling a weighty generate load? Consider databases that could cope with high compose throughput, as well as party-based information storage devices like Apache Kafka (for temporary information streams).
It’s also wise to Consider in advance. You might not have to have advanced scaling attributes now, but selecting a database that supports them usually means you received’t need to switch later.
Use indexing to speed up queries. Steer clear of needless joins. Normalize or denormalize your info dependant upon your entry designs. And generally keep track of database efficiency as you develop.
In brief, the correct database depends upon your app’s structure, velocity requires, and how you anticipate it to grow. Take time to select sensibly—it’ll help save a great deal of difficulties later on.
Optimize Code and Queries
Fast code is essential to scalability. As your application grows, each individual smaller hold off adds up. Poorly penned code or unoptimized queries can decelerate functionality and overload your program. That’s why it’s crucial to build economical logic from the beginning.
Start out by composing thoroughly clean, simple code. Stay clear of repeating logic and take away anything at all unnecessary. Don’t pick the most sophisticated Answer if a straightforward just one operates. Maintain your functions small, targeted, and straightforward to test. Use profiling tools to search out bottlenecks—areas where your code can take as well extensive to run or makes use of too much memory.
Upcoming, take a look at your databases queries. These frequently gradual issues down in excess of the code itself. Ensure that Each and every question only asks for the data you truly want. Stay clear of Pick *, which fetches all the things, and as an alternative find certain fields. Use indexes to hurry up lookups. And avoid undertaking a lot of joins, Particularly throughout huge tables.
When you discover precisely the same data getting asked for many times, use caching. Shop the outcome quickly using equipment like Redis or Memcached this means you don’t need to repeat highly-priced operations.
Also, batch your database operations if you can. In lieu of updating a row one by one, update them in groups. This cuts down on overhead and helps make your app far more economical.
Make sure to test with big datasets. Code and queries that get the job done fine with 100 records may well crash whenever they have to manage one million.
Briefly, scalable applications are speedy applications. Keep the code limited, your queries lean, and use caching when needed. These steps support your software keep clean and responsive, even as the load increases.
Leverage Load Balancing and Caching
As your app grows, it has to take care of more users and much more visitors. If every thing goes via one particular server, it is going to speedily become a bottleneck. That’s in which load balancing and caching are available in. These two tools help keep the application rapidly, steady, and scalable.
Load balancing spreads incoming site visitors across multiple servers. As opposed to a single server performing all of the work, the load balancer routes buyers to unique servers determined by availability. This implies no single server receives overloaded. If one particular server goes down, the load balancer can deliver visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily based answers from AWS and Google Cloud make this easy to arrange.
Caching is about storing knowledge temporarily so it might be reused speedily. When customers ask for precisely the same info all over again—like an item page or maybe a profile—you don’t must fetch it through the database anytime. You'll be able to provide it from your cache.
There's two widespread kinds of caching:
one. Server-side caching (like Redis or Memcached) merchants information in memory for rapid accessibility.
two. Client-aspect caching (like browser caching or CDN caching) retailers static data files close to the person.
Caching minimizes databases load, improves pace, and will make your app additional effective.
Use caching for things which don’t change generally. And usually be sure your cache is updated when knowledge does improve.
In brief, load balancing and caching are uncomplicated but potent instruments. Together, they help your app tackle much more end users, continue to be quick, and Get well from complications. If you plan to improve, you need the two.
Use Cloud and Container Instruments
To build scalable applications, you may need instruments that permit your application develop very easily. That’s wherever cloud platforms and containers are available. They offer you flexibility, decrease setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon Web Providers (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and expert services as you would like them. You don’t really have to buy hardware or guess long term capacity. When site visitors raises, it is possible to incorporate far more methods with just a couple clicks or automatically using auto-scaling. When visitors drops, you are able to scale down to save money.
These platforms also offer services like managed databases, storage, load balancing, and security tools. You can deal with setting up your application as an alternative to taking care of infrastructure.
Containers are A different here essential Device. A container packages your app and every thing it needs to operate—code, libraries, options—into a single unit. This can make it uncomplicated to move your app involving environments, from the laptop to the cloud, without the need of surprises. Docker is the most well-liked Instrument for this.
Once your application makes use of several containers, tools like Kubernetes assist you take care of them. Kubernetes handles deployment, scaling, and recovery. If a person aspect of one's application crashes, it restarts it routinely.
Containers also allow it to be straightforward to individual parts of your application into providers. You can update or scale sections independently, which can be perfect for functionality and dependability.
To put it briefly, working with cloud and container resources usually means you'll be able to scale fast, deploy simply, and recover speedily when problems come about. If you would like your application to grow with no restrictions, commence applying these resources early. They help save time, reduce chance, and assist you to keep centered on developing, not repairing.
Observe Every thing
In case you don’t monitor your application, you gained’t know when points go wrong. Monitoring will help the thing is how your application is carrying out, place difficulties early, and make better decisions as your app grows. It’s a essential A part of constructing scalable devices.
Start by tracking fundamental metrics like CPU utilization, memory, disk Place, and reaction time. These show you how your servers and products and services are performing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you accumulate and visualize this facts.
Don’t just observe your servers—monitor your app too. Keep an eye on how long it will take for consumers to load webpages, how frequently glitches transpire, and where by they manifest. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on within your code.
Build alerts for significant challenges. One example is, In the event your reaction time goes earlier mentioned a limit or even a support goes down, you need to get notified instantly. This assists you fix challenges speedy, generally before users even see.
Checking is additionally helpful when you make variations. For those who deploy a different characteristic and see a spike in faults or slowdowns, you may roll it back again before it results in true injury.
As your application grows, website traffic and info improve. Without the need of monitoring, you’ll miss indications of problems until it’s too late. But with the ideal equipment in place, you keep in control.
To put it briefly, monitoring allows you maintain your application trustworthy and scalable. It’s not pretty much spotting failures—it’s about understanding your technique and making sure it really works well, even under pressure.
Final Feelings
Scalability isn’t just for massive companies. Even modest apps need to have a solid foundation. By coming up with thoroughly, optimizing wisely, and using the ideal resources, you may Develop applications that mature easily devoid of breaking under pressure. Commence smaller, Believe massive, and Establish intelligent.